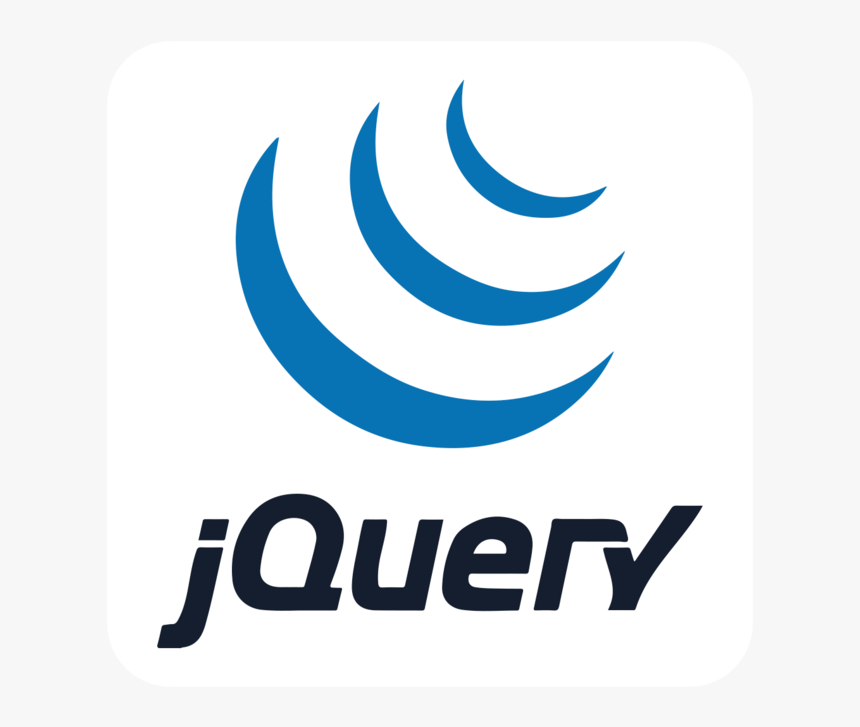
test1.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
/* <body onload=sendIt()> */
/* 자바스크립트에서는 자료형이 없다
변수 onload에 함수를 넣었다 */
/* window.onload = function() {
alert("환영!!")
}
window.onload = function() {
alert("진짜환영!!")
} */
/* 순수한자바스크립트는 진짜환영이 안보이고 JQuery는 진짜환영이 보인다. */
$(document).ready(function(){
alert("환영")
})
$(document).ready(function(){
alert("진짜환영")
})
/* 위문장을 아래문장으로 함축시킬수있다. */
$(function(){
$(document.body).css("background","pink");
})
/* body에 삽입 */
$(function(){
$("<div><p>제이쿼리</p></div>").appendTo("body");
})
</script>
</head>
<body>
</body>
</html>
$(document).ready(function()
창이 열리면서 자동으로 호출되는 함수
document는 DOM객체의 최상위 객체이다.
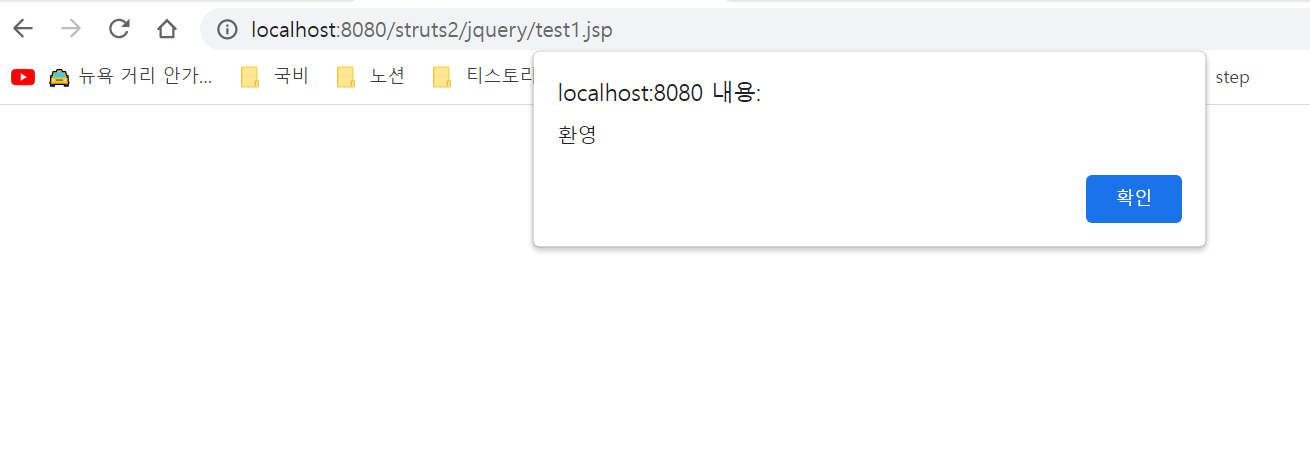
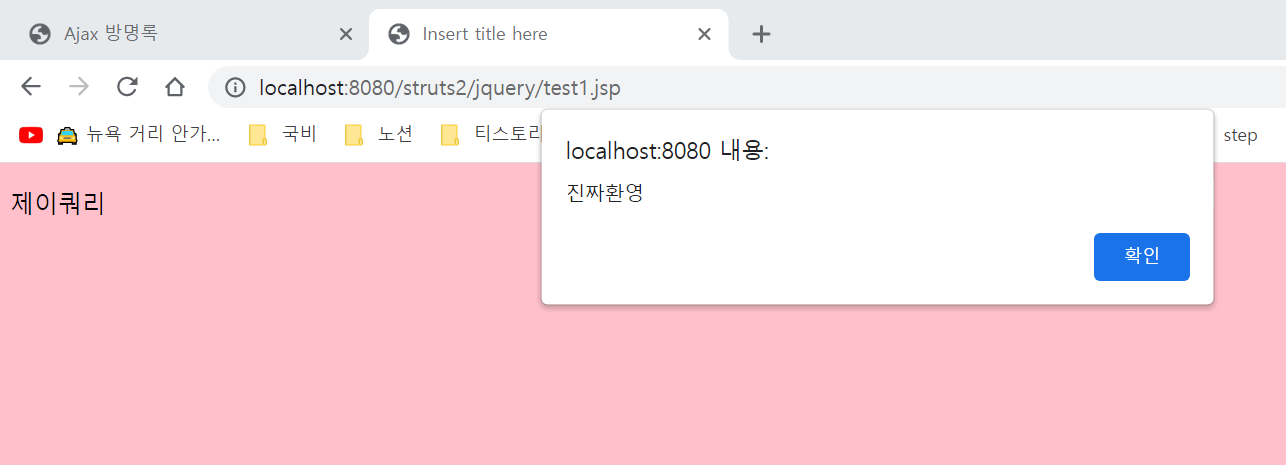
test2.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
$(function(){
$("#btnOK").click(function() { /* btnOK를 클릭하면 함수를 실행해라 */
var msg = $("#userName").val() /* userName의 value값 읽어와라 */
msg += "\r\n" + $("input:radio[name=gender]:checked").val() /* input 박스중에 radio라는 곳에 name이 gender의 값을 가져와라 checked되어있는 값의 value를 읽어와라 */
msg += "\r\n" + $("#hobby").val().join("|") /* hobby값을 가져올때 |구분하여 가져와라 */
alert(msg)
})
})
/* form이 없을때 id로 접근 (JavaScript) */
function result() {
var msg = document.getElementById("userName").value /* 하나의 요소에 id값으로 찾아간다 */
alert(msg)
}
</script>
</head>
<body>
<!-- css에서 id로 적으면 제이쿼리로 읽어낸다. id는 고유이름 -->
이름: <input type="text" id="userName"/><br/>
성별: <input type="radio" id="gender1" value="M" name="gender">남자
<input type="radio" id="gender2" value="F" name="gender">여자<br/><br/>
취미: <select id="hobby" multiple="multiple">
<option value="여행">여행</option>
<option value="영화">영화</option>
<option value="운동">운동</option>
<option value="게임">게임</option>
</select>
<br/><br/>
jQuery: <input type="button" value="확인" id="btnOK"/><br/>
Javascript: <input type="button" value="확인" onclick="result();"/><br/>
</body>
</html>
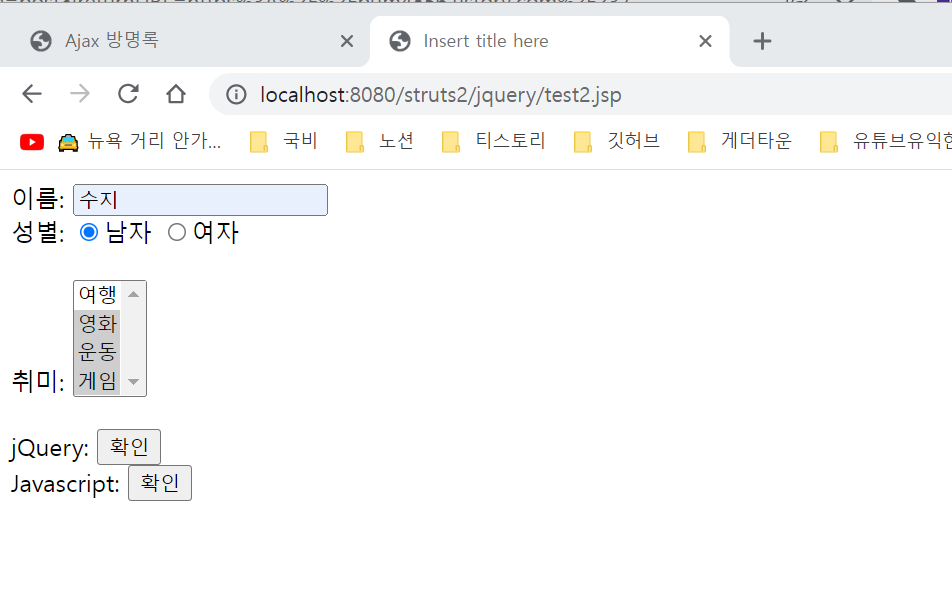
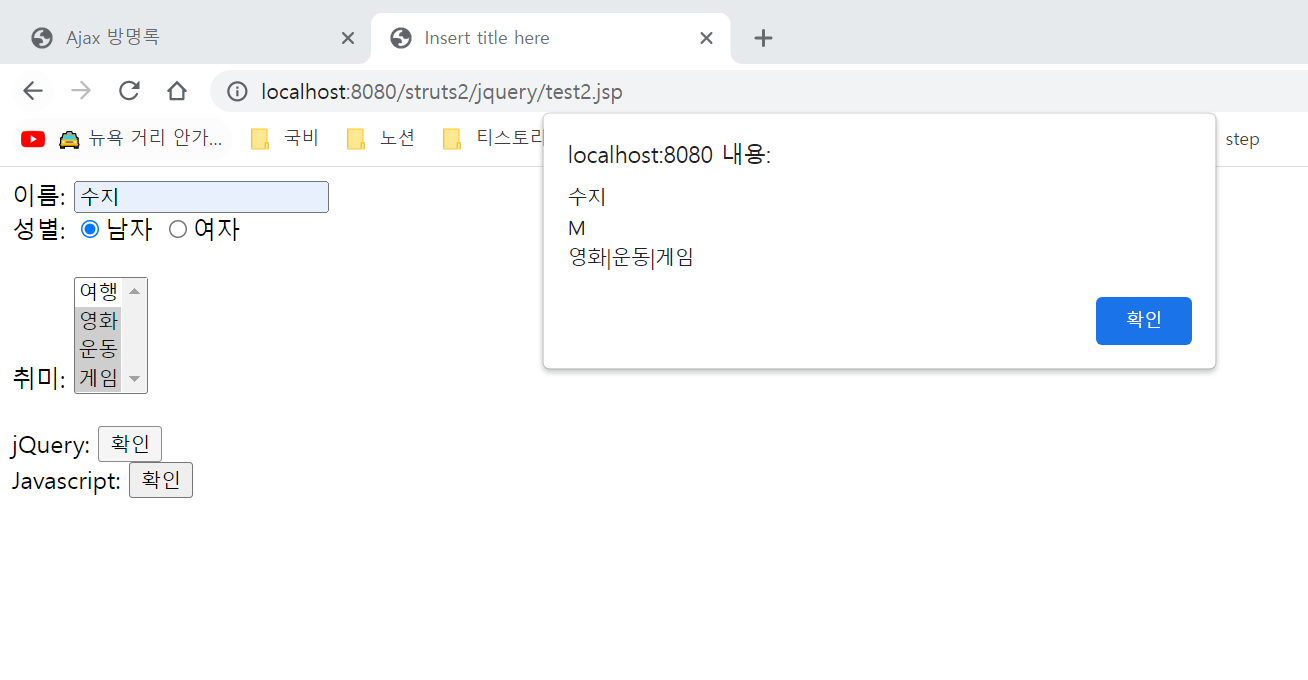
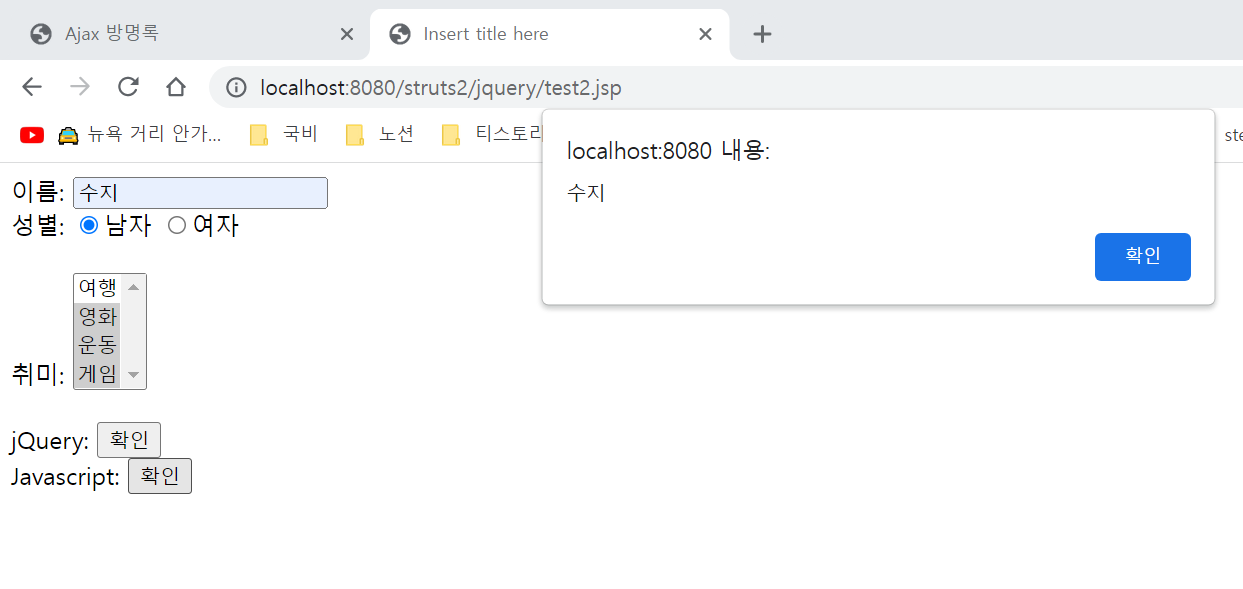
test3.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="<%=cp %>/data/css/jquery-ui.min.css"/>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-ui.min.js"></script>
<title>Insert title here</title>
<!-- 제이쿼리 UI : 디자인을 하지않아도 디자인이 보인다. -->
<script type="text/javascript">
$(function() {
$("#dialog").hide(); /* dialog를 기본적으로 숨기고 */
$("#btn1").click(function() { /* btn1버튼을 누르면 dialog 창을 보여준다. */
$("#dialog").dialog();
})
$("#btn2").click(function() {
$("#dialog").dialog({ /* dialog창을 띄울때 내가 원하는 무언가할것이다. */
height:240,
width:300,
model:true /* 모달 : 앞에 있는 창을 해결해야 넘어간다. */
})
})
/* 외부 ex.jsp 띄우기 */
$("#btn3").click(function() {
$("<div>").dialog({
modal:true,
open:function(){
$(this).load("ex.jsp") /* this 자기자신을 띄어라 */
},
height:400,
width:400,
title:"외부 파일 창 띄우기"
})
})
})
</script>
</head>
<body>
<div id="dialog" title="우편번호 검색">
<p>동을 입력하세요</p>
<p><input type="text"/></p>
</div>
<!-- ui가 들어가서 자동으로 적용 -->
<div>
<input type="button" value="모달리스" id="btn1"/><br/>
<input type="button" value="모달" id="btn2"/><br/>
<input type="button" value="창띄우기" id="btn3"/><br/>
</div>
</body>
</html>
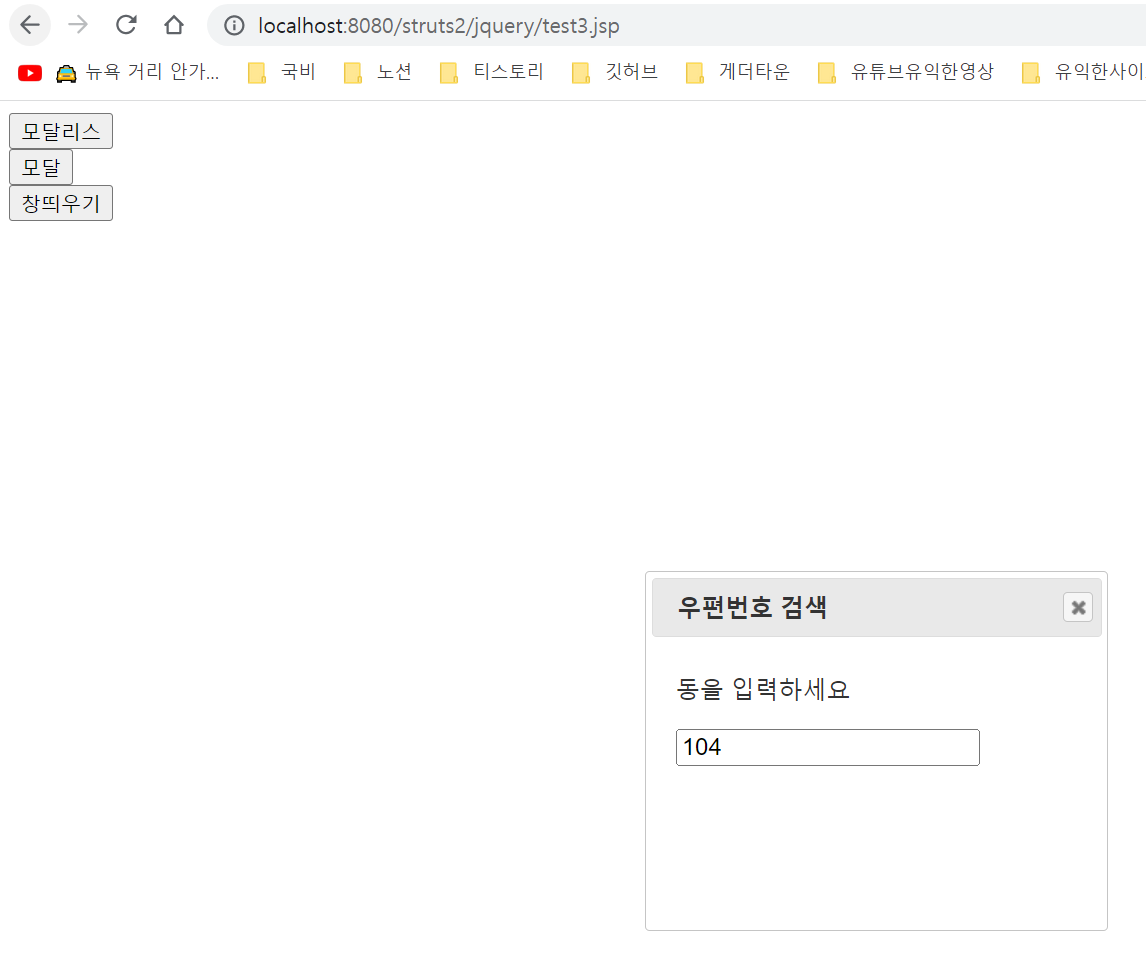
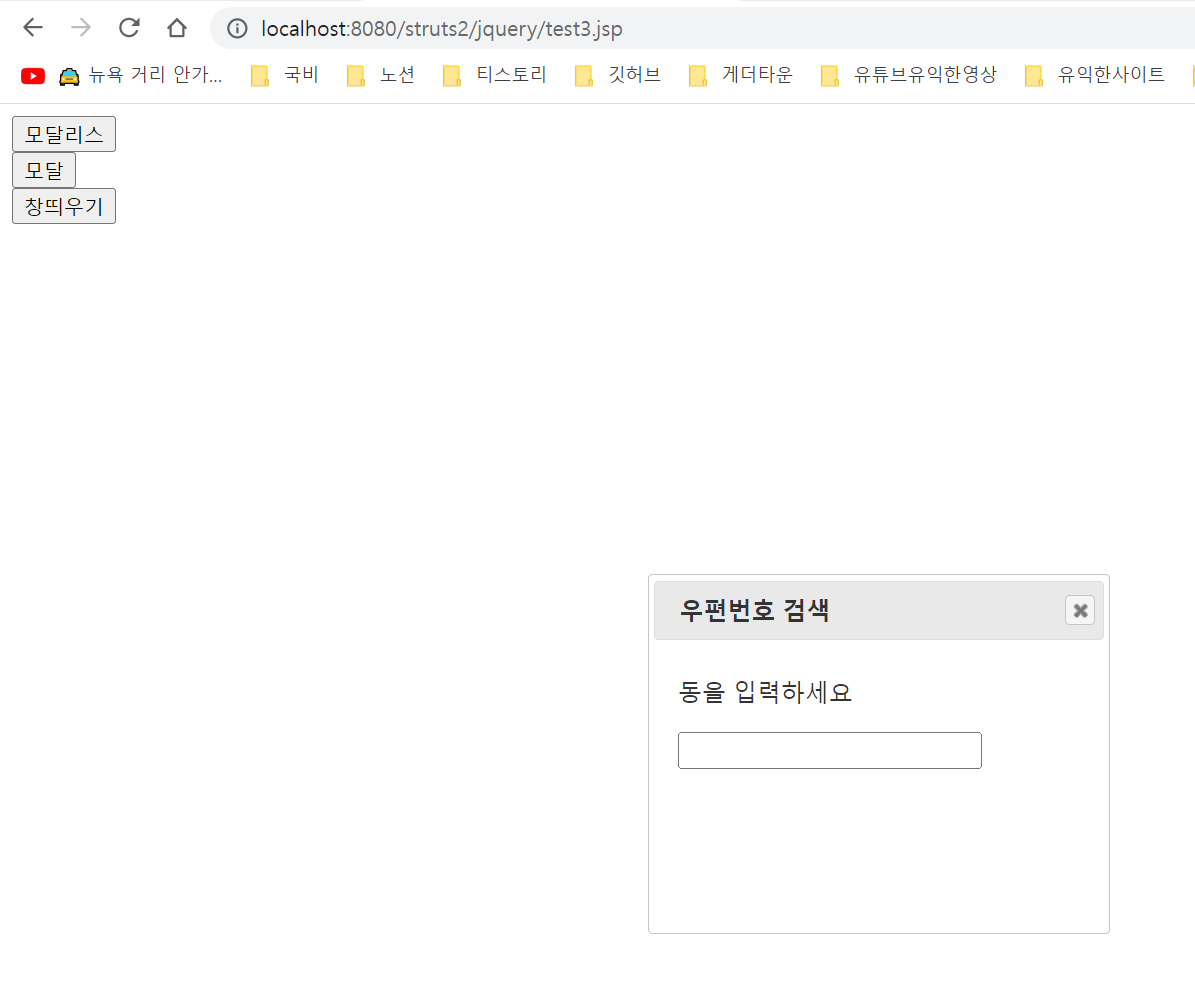
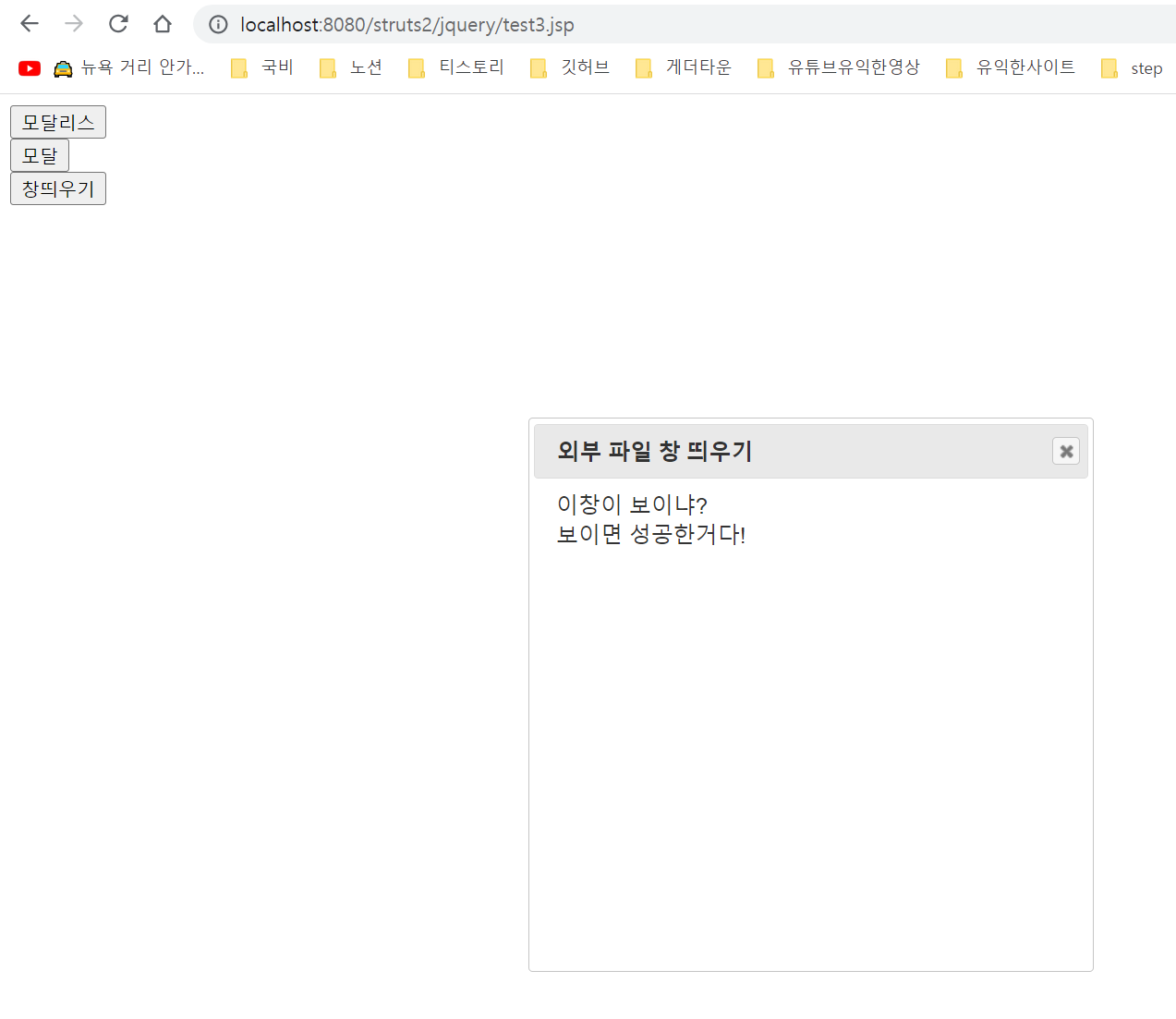
test4.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="<%=cp %>/data/css/jquery-ui.min.css"/>
<style type="text/css">
body{ font: 62.5% "굴림", sans-serif; margin: 50px;}
ul#icons {margin: 0; padding: 0;}
ul#icons li {margin: 2px; position: relative; padding: 4px 0; cursor: pointer; float: left; list-style: none;}
ul#icons span.ui-icon {float: left; margin: 0 4px;}
#container { width: 300px;}
</style>
<!-- 제이쿼리ui 페이지에서 소스코드를 가져올수있다.(파일경로가필요없어짐) - CDN방식이라고 한다. -->
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<title>Insert title here</title>
<script type="text/javascript">
$(function() {
/* #의의미는 호출 */
$("#container").tabs(); /* 탭을 호출한다. */
})
</script>
</head>
<body>
<div id="container">
<ul>
<li><a href="#f1">첫번째</a></li> <!-- #사용자정의 -->
<li><a href="#f2">두번째</a></li>
<li><a href="#f3">세번째</a></li>
</ul>
<div id="f1">
나는 일등이다
</div>
<div id="f2">
나는 이등이다
</div>
<div id="f3">
나는 삼등이다
</div>
</div>
</body>
</html>
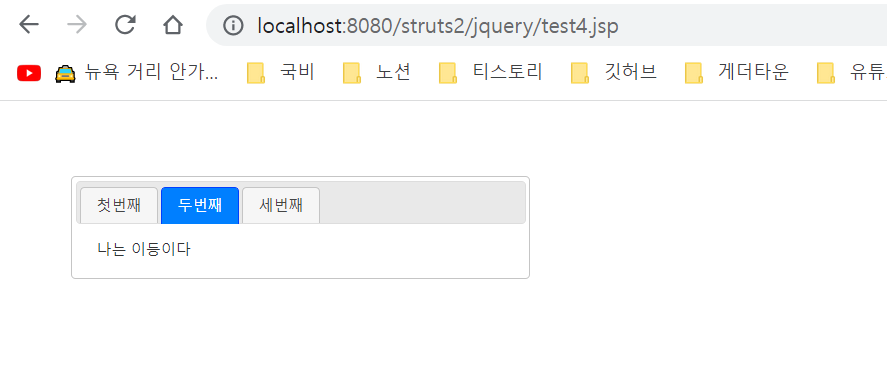
test5.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<!-- https://jqueryui.com/droppable/ 소스를 가져와서 실행 -->
<!-- 실시간 대화창만들때 많이 쓰인다. -->
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Droppable - Default functionality</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
#draggable { width: 100px; height: 100px; padding: 0.5em; float: left; margin: 10px 10px 10px 0; }
#droppable { width: 150px; height: 150px; padding: 0.5em; float: left; margin: 10px; }
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<script>
$(function() {
$( "#draggable" ).draggable();
$( "#droppable" ).droppable({
drop: function( event, ui ) {
$( this )
.addClass( "ui-state-highlight" )
.find( "p" )
.html( "Dropped!" );
}
});
} );
</script>
</head>
<body>
<div id="draggable" class="ui-widget-content">
<p>Drag me to my target</p>
</div>
<div id="droppable" class="ui-widget-header">
<p>Drop here</p>
</div>
</body>
</html>
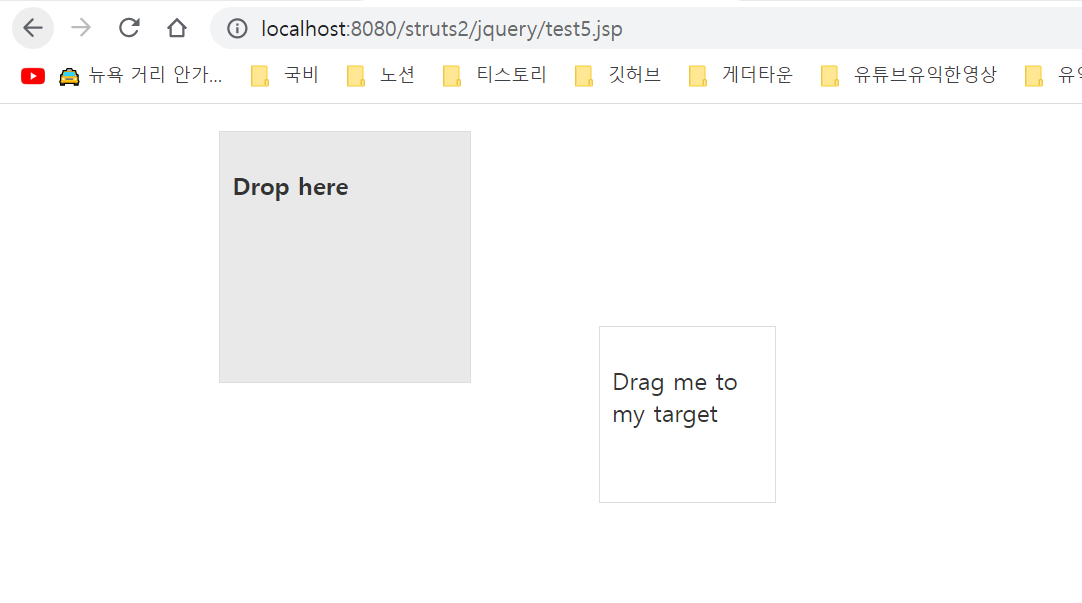
'FRONTEND > Jquery' 카테고리의 다른 글
[Jquery] Jquery란? (0) | 2022.03.15 |
---|
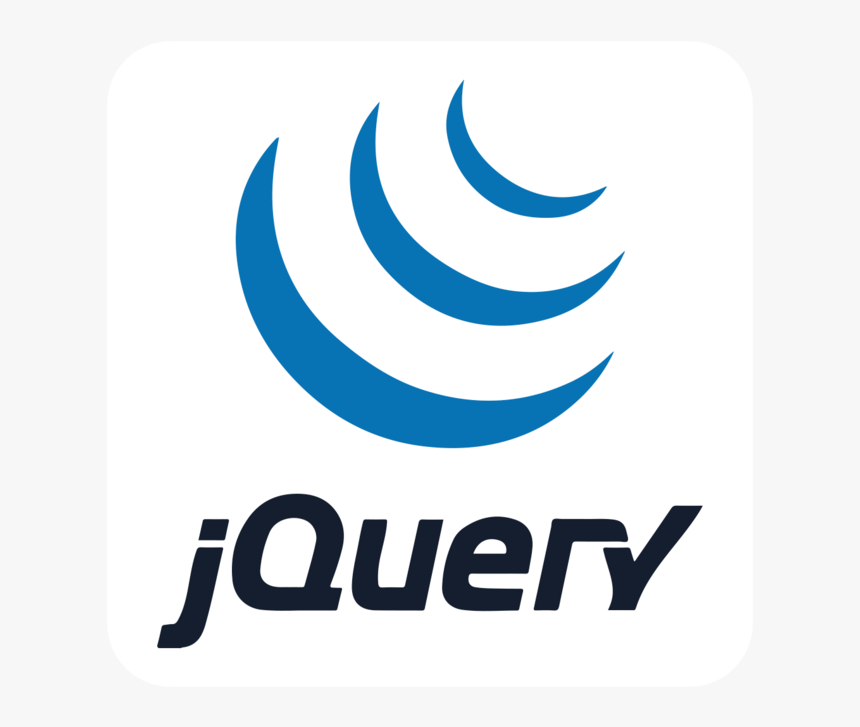
test1.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
/* <body onload=sendIt()> */
/* 자바스크립트에서는 자료형이 없다
변수 onload에 함수를 넣었다 */
/* window.onload = function() {
alert("환영!!")
}
window.onload = function() {
alert("진짜환영!!")
} */
/* 순수한자바스크립트는 진짜환영이 안보이고 JQuery는 진짜환영이 보인다. */
$(document).ready(function(){
alert("환영")
})
$(document).ready(function(){
alert("진짜환영")
})
/* 위문장을 아래문장으로 함축시킬수있다. */
$(function(){
$(document.body).css("background","pink");
})
/* body에 삽입 */
$(function(){
$("<div><p>제이쿼리</p></div>").appendTo("body");
})
</script>
</head>
<body>
</body>
</html>
$(document).ready(function()
창이 열리면서 자동으로 호출되는 함수
document는 DOM객체의 최상위 객체이다.
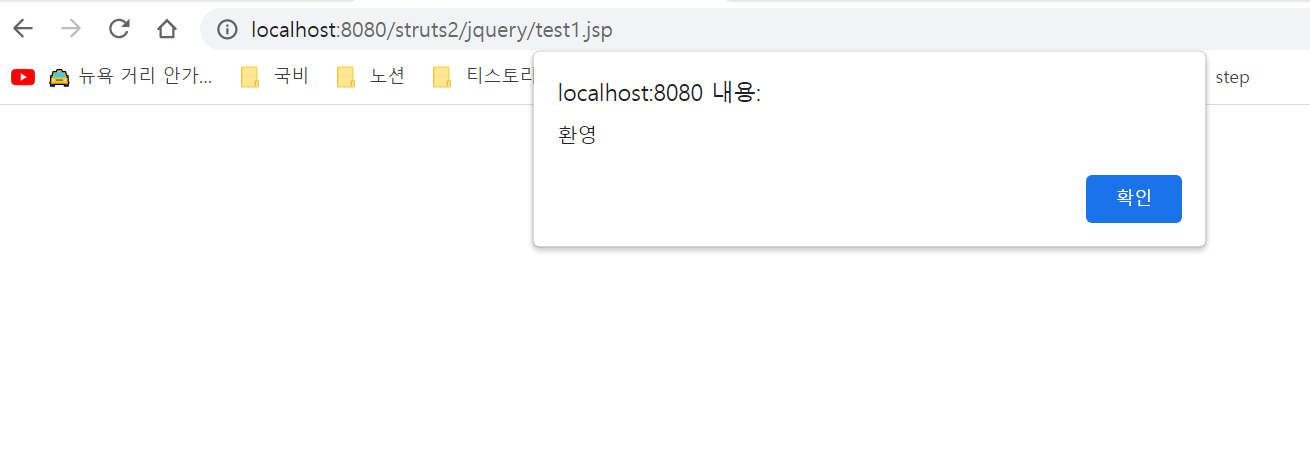
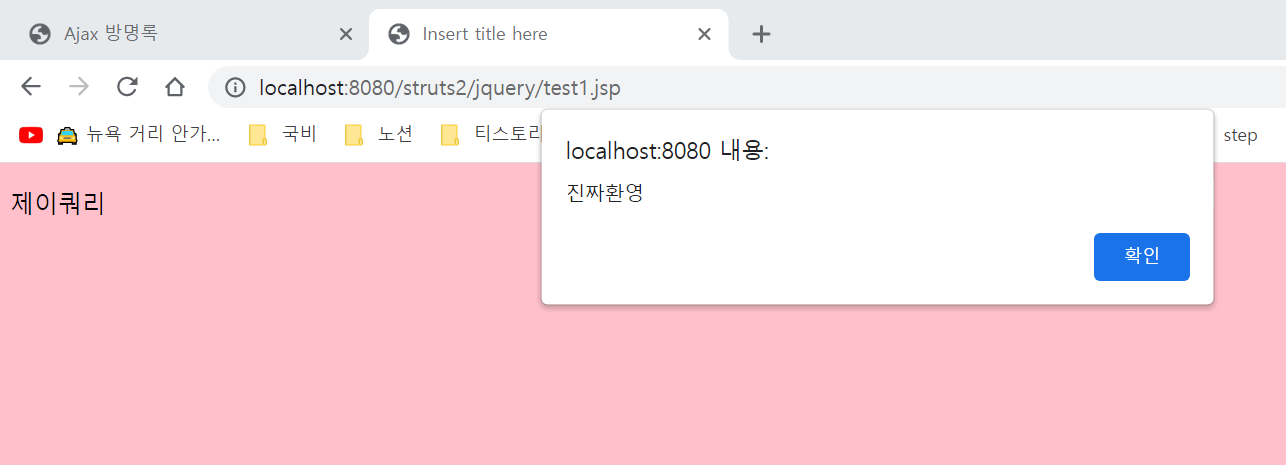
test2.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript">
$(function(){
$("#btnOK").click(function() { /* btnOK를 클릭하면 함수를 실행해라 */
var msg = $("#userName").val() /* userName의 value값 읽어와라 */
msg += "\r\n" + $("input:radio[name=gender]:checked").val() /* input 박스중에 radio라는 곳에 name이 gender의 값을 가져와라 checked되어있는 값의 value를 읽어와라 */
msg += "\r\n" + $("#hobby").val().join("|") /* hobby값을 가져올때 |구분하여 가져와라 */
alert(msg)
})
})
/* form이 없을때 id로 접근 (JavaScript) */
function result() {
var msg = document.getElementById("userName").value /* 하나의 요소에 id값으로 찾아간다 */
alert(msg)
}
</script>
</head>
<body>
<!-- css에서 id로 적으면 제이쿼리로 읽어낸다. id는 고유이름 -->
이름: <input type="text" id="userName"/><br/>
성별: <input type="radio" id="gender1" value="M" name="gender">남자
<input type="radio" id="gender2" value="F" name="gender">여자<br/><br/>
취미: <select id="hobby" multiple="multiple">
<option value="여행">여행</option>
<option value="영화">영화</option>
<option value="운동">운동</option>
<option value="게임">게임</option>
</select>
<br/><br/>
jQuery: <input type="button" value="확인" id="btnOK"/><br/>
Javascript: <input type="button" value="확인" onclick="result();"/><br/>
</body>
</html>
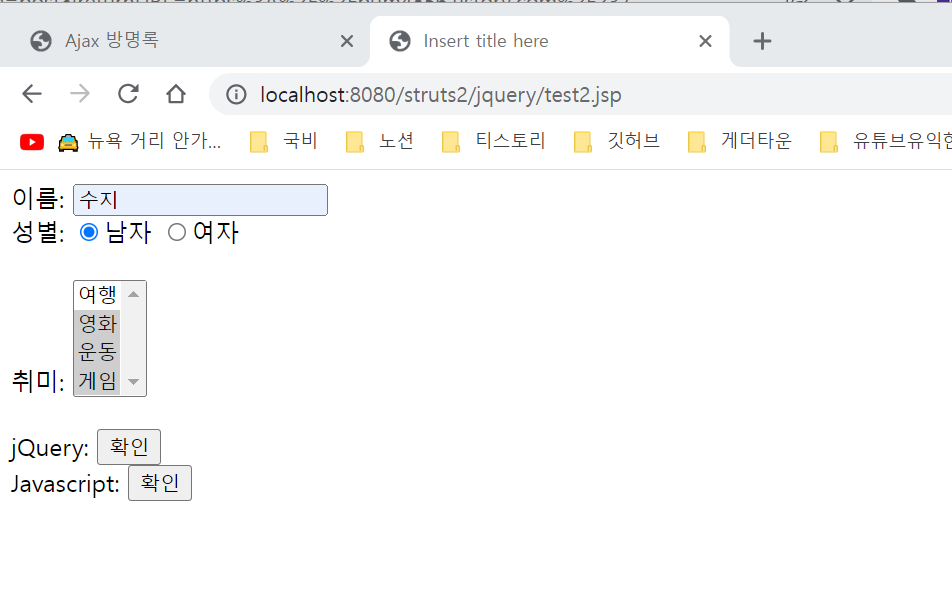
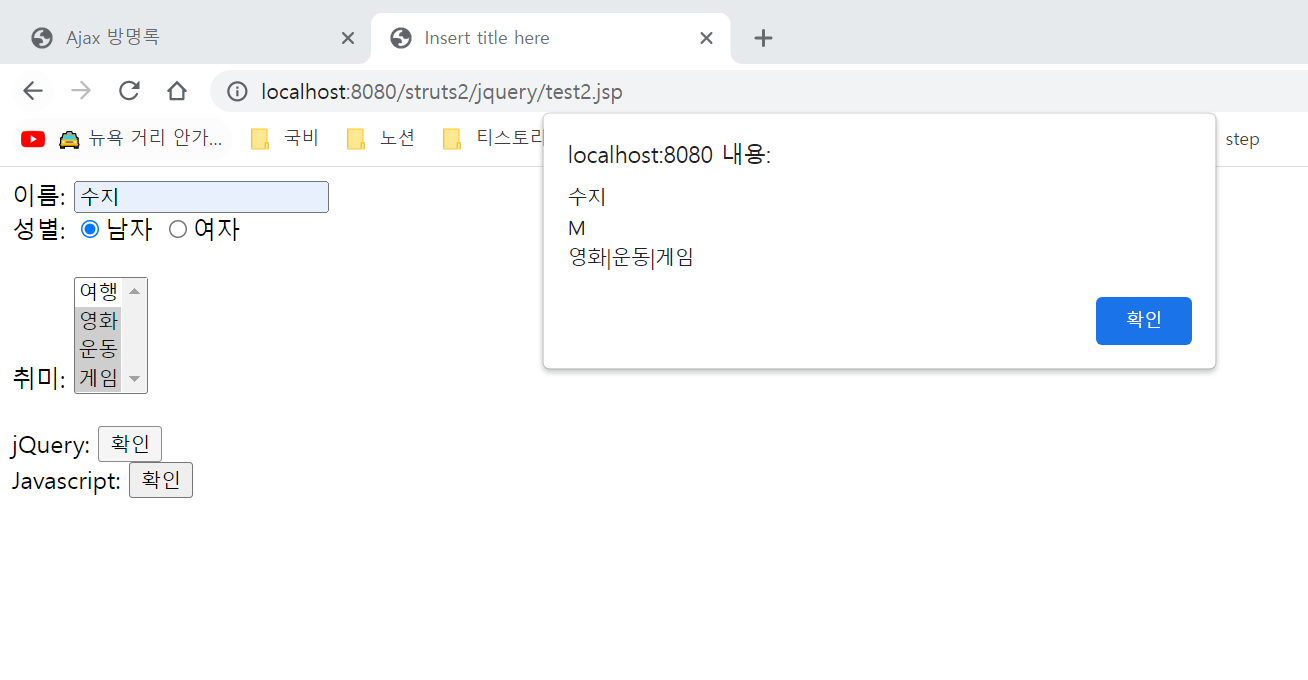
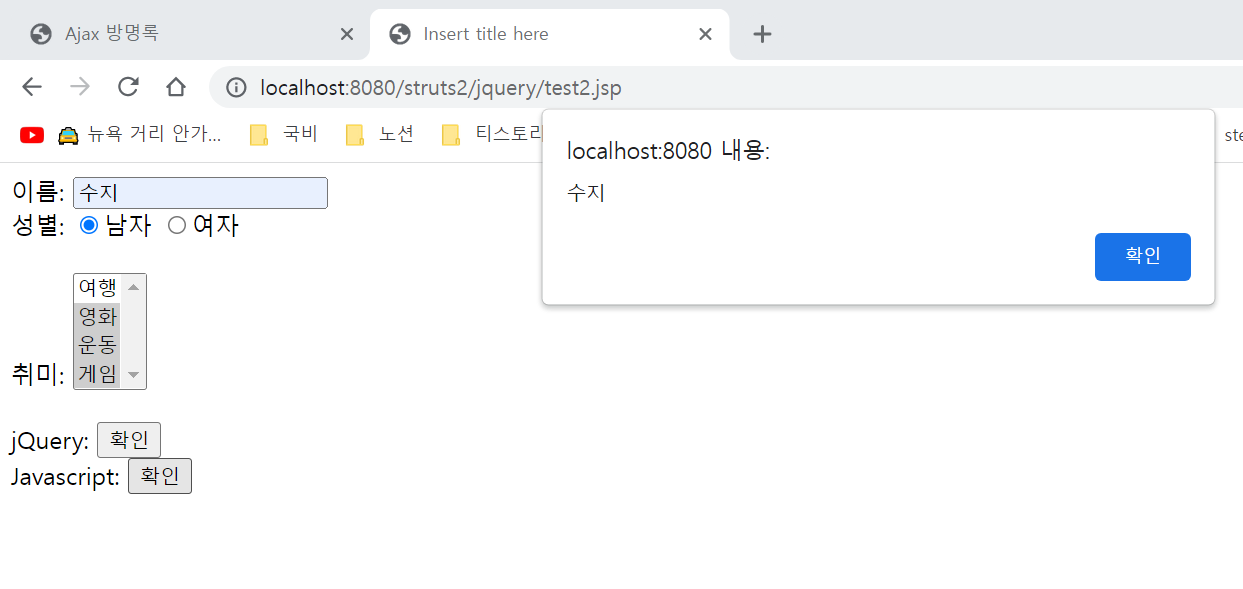
test3.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="<%=cp %>/data/css/jquery-ui.min.css"/>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-3.1.1.min.js"></script>
<script type="text/javascript" src="<%=cp%>/data/js/jquery-ui.min.js"></script>
<title>Insert title here</title>
<!-- 제이쿼리 UI : 디자인을 하지않아도 디자인이 보인다. -->
<script type="text/javascript">
$(function() {
$("#dialog").hide(); /* dialog를 기본적으로 숨기고 */
$("#btn1").click(function() { /* btn1버튼을 누르면 dialog 창을 보여준다. */
$("#dialog").dialog();
})
$("#btn2").click(function() {
$("#dialog").dialog({ /* dialog창을 띄울때 내가 원하는 무언가할것이다. */
height:240,
width:300,
model:true /* 모달 : 앞에 있는 창을 해결해야 넘어간다. */
})
})
/* 외부 ex.jsp 띄우기 */
$("#btn3").click(function() {
$("<div>").dialog({
modal:true,
open:function(){
$(this).load("ex.jsp") /* this 자기자신을 띄어라 */
},
height:400,
width:400,
title:"외부 파일 창 띄우기"
})
})
})
</script>
</head>
<body>
<div id="dialog" title="우편번호 검색">
<p>동을 입력하세요</p>
<p><input type="text"/></p>
</div>
<!-- ui가 들어가서 자동으로 적용 -->
<div>
<input type="button" value="모달리스" id="btn1"/><br/>
<input type="button" value="모달" id="btn2"/><br/>
<input type="button" value="창띄우기" id="btn3"/><br/>
</div>
</body>
</html>
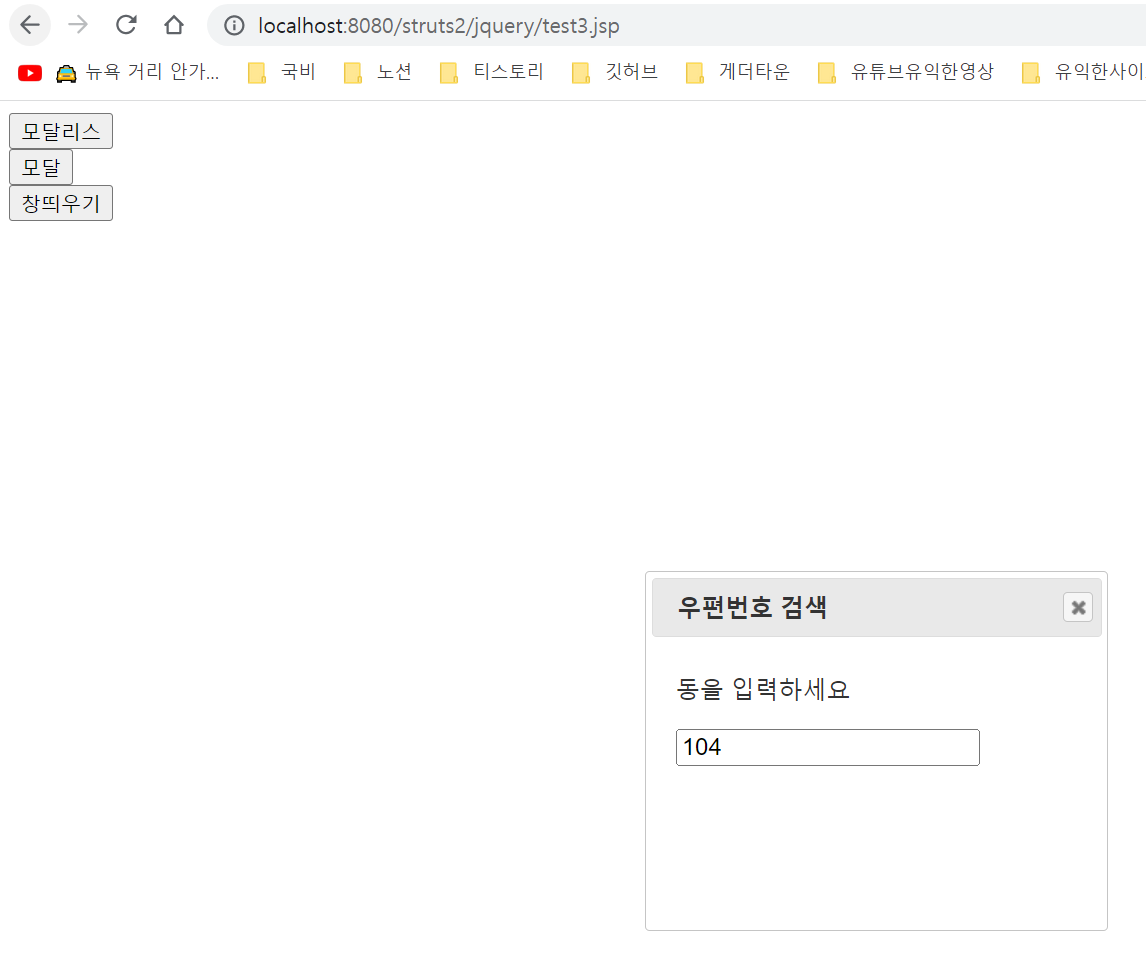
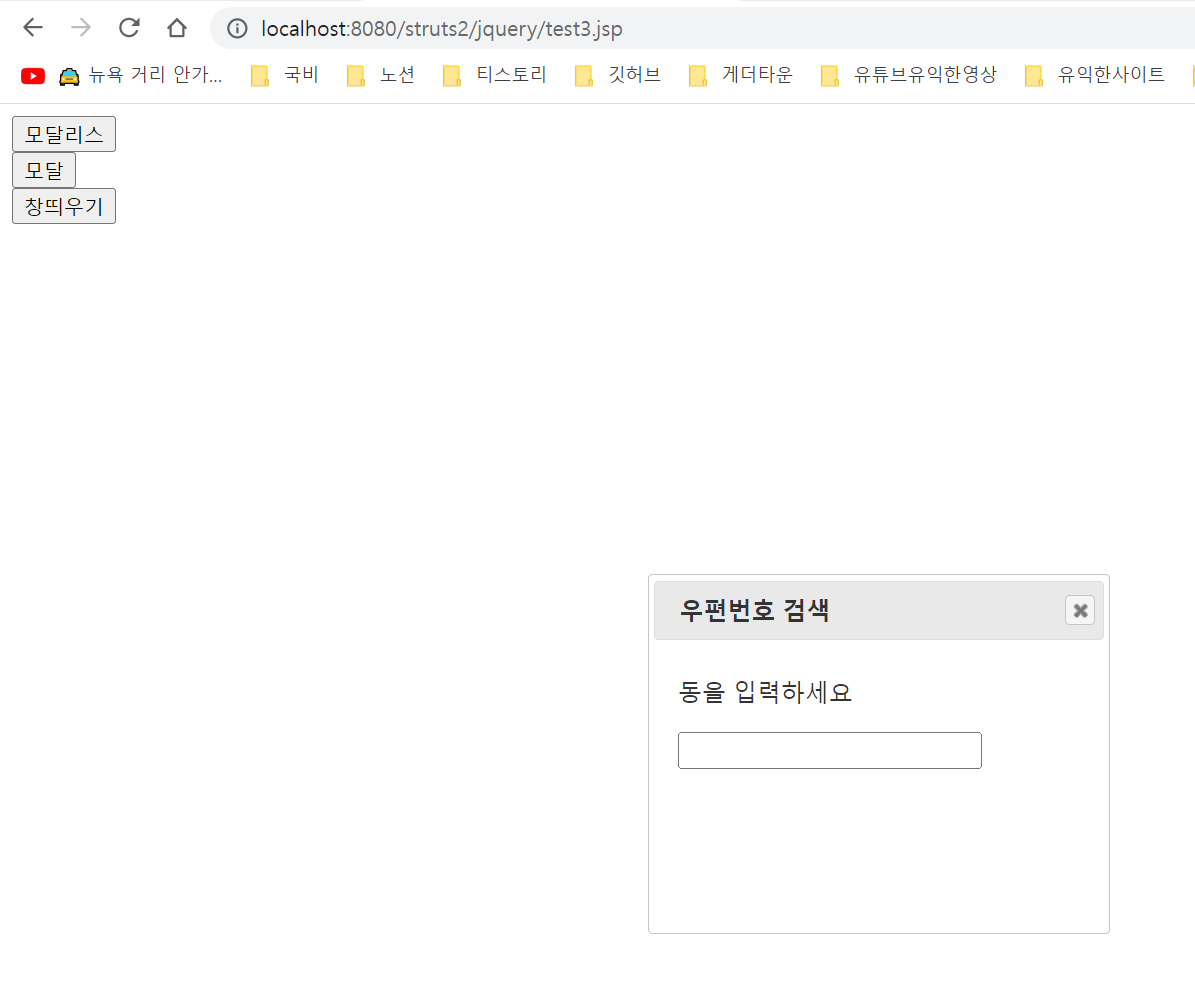
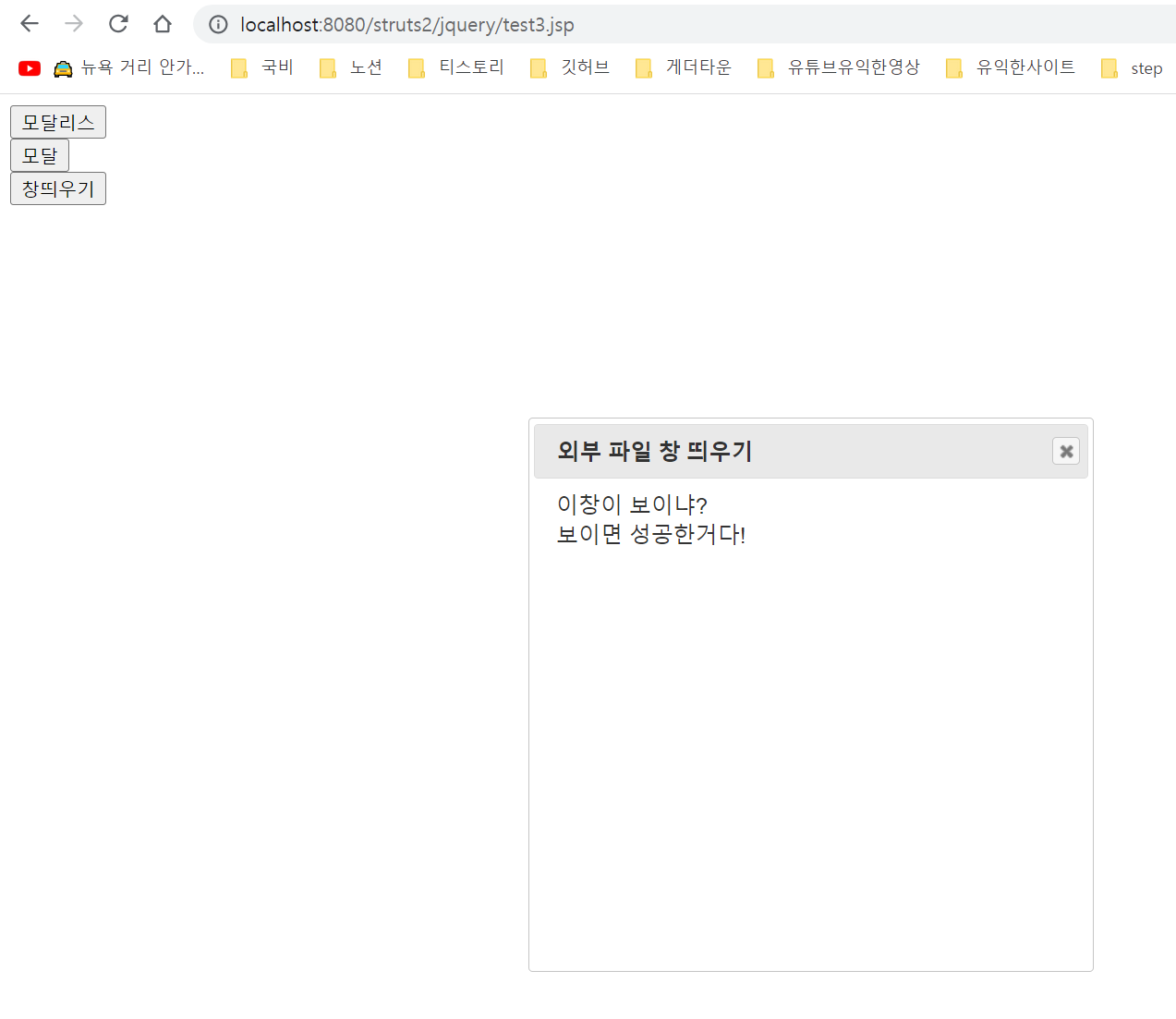
test4.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<link rel="stylesheet" type="text/css" href="<%=cp %>/data/css/jquery-ui.min.css"/>
<style type="text/css">
body{ font: 62.5% "굴림", sans-serif; margin: 50px;}
ul#icons {margin: 0; padding: 0;}
ul#icons li {margin: 2px; position: relative; padding: 4px 0; cursor: pointer; float: left; list-style: none;}
ul#icons span.ui-icon {float: left; margin: 0 4px;}
#container { width: 300px;}
</style>
<!-- 제이쿼리ui 페이지에서 소스코드를 가져올수있다.(파일경로가필요없어짐) - CDN방식이라고 한다. -->
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<title>Insert title here</title>
<script type="text/javascript">
$(function() {
/* #의의미는 호출 */
$("#container").tabs(); /* 탭을 호출한다. */
})
</script>
</head>
<body>
<div id="container">
<ul>
<li><a href="#f1">첫번째</a></li> <!-- #사용자정의 -->
<li><a href="#f2">두번째</a></li>
<li><a href="#f3">세번째</a></li>
</ul>
<div id="f1">
나는 일등이다
</div>
<div id="f2">
나는 이등이다
</div>
<div id="f3">
나는 삼등이다
</div>
</div>
</body>
</html>
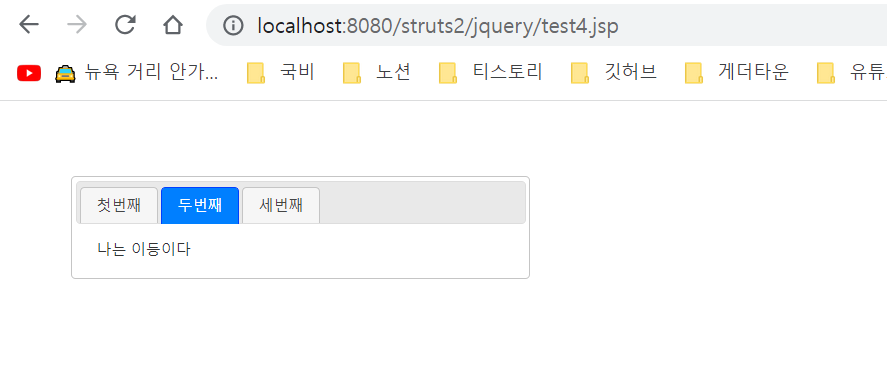
test5.jsp
<%@ page contentType="text/html; charset=UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%
request.setCharacterEncoding("UTF-8");
String cp = request.getContextPath();
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<!-- https://jqueryui.com/droppable/ 소스를 가져와서 실행 -->
<!-- 실시간 대화창만들때 많이 쓰인다. -->
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>jQuery UI Droppable - Default functionality</title>
<link rel="stylesheet" href="//code.jquery.com/ui/1.13.1/themes/base/jquery-ui.css">
<link rel="stylesheet" href="/resources/demos/style.css">
<style>
#draggable { width: 100px; height: 100px; padding: 0.5em; float: left; margin: 10px 10px 10px 0; }
#droppable { width: 150px; height: 150px; padding: 0.5em; float: left; margin: 10px; }
</style>
<script src="https://code.jquery.com/jquery-3.6.0.js"></script>
<script src="https://code.jquery.com/ui/1.13.1/jquery-ui.js"></script>
<script>
$(function() {
$( "#draggable" ).draggable();
$( "#droppable" ).droppable({
drop: function( event, ui ) {
$( this )
.addClass( "ui-state-highlight" )
.find( "p" )
.html( "Dropped!" );
}
});
} );
</script>
</head>
<body>
<div id="draggable" class="ui-widget-content">
<p>Drag me to my target</p>
</div>
<div id="droppable" class="ui-widget-header">
<p>Drop here</p>
</div>
</body>
</html>
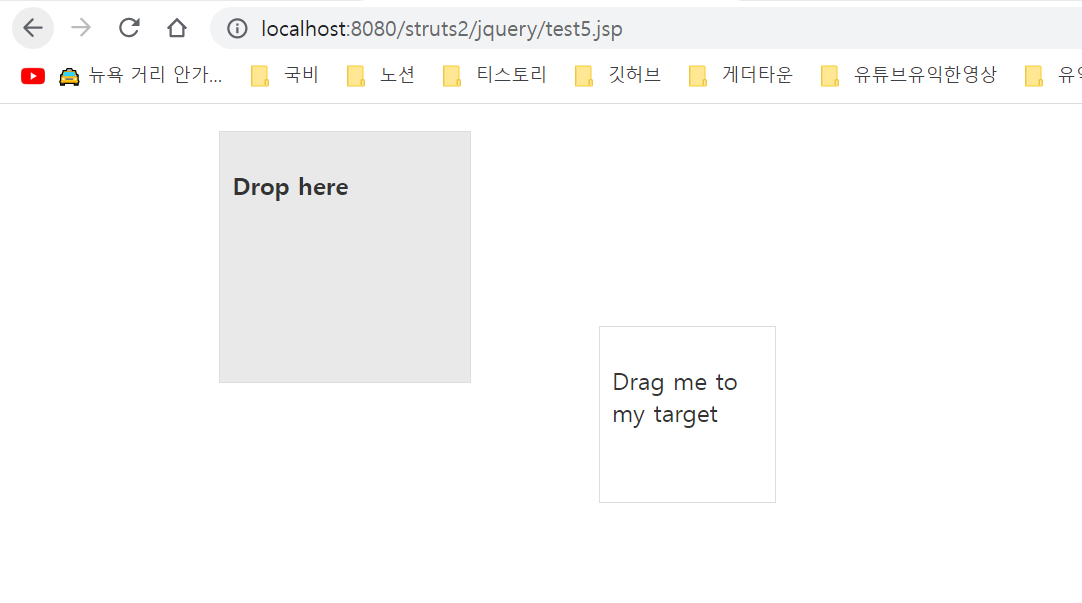
'FRONTEND > Jquery' 카테고리의 다른 글
[Jquery] Jquery란? (0) | 2022.03.15 |
---|